Run Node.js application in Docker & deploy it to Microsoft Azure
Utilising cloud services make it easy to deploy Node.js applications online, without the need to setup an own virtual private server (VPS) and install and configure the needed operating system and/or runtimes in it. It’s also an important tool for the realisation of web solutions based on micro services.
In this article, I’ll show how to run a minimal Node.js application inside Docker and deploy it to Microsoft Azure using the Azure Container Registry and Azure App Services.
Prerequisite
- locally running Node.js installation
- locally running Docker desktop installation
- an Microsoft Azure account
1. Create an minimal Node.js application
First we need to create a minimal Node.js application. For this, you can use the Node.js boilerplate found in my GitHub repository (https://github.com/krga/nodejs-minimal) or create the following two files an empty directory.
index.js:
// index.js
const express = require("express"); const app = express(); // get the PORT environment variable or user port 3000 as default const PORT = process.env.PORT || 3000; // register GET route at / app.get("/", (req, res) => { res.send("Hello from docker!"); }); // start HTTP server at the port defined in const PORT app.listen(PORT, () => { console.log(`minimal node.js boilerplate app listening on port ${PORT}, open http://localhost:${PORT}`); });
package.json:
{ "name": "nodejs-minimal", "version": "1.0.0", "description": " a minimal node.js boilerplate with configurable ports", "main": "index.js", "scripts": { "start": "node index.js" }, "author": "Kristian Gavran", "license": "MIT", "dependencies": { "express": "^4.17.1" } }
These are the minimal two files needed to run a simple Node.js app and to be able to install any runtime dependencies. We will test run the application locally, and ensure that it works as expected by:
- installing any dependencies defined inside of package.json:
user@local:~# npm install
npm WARN nodejs-minimal@1.0.0 No repository field.
added 50 packages from 37 contributors and audited 50 packages in 1.145s
found 0 vulnerabilities
- run the Node.js app and open it in the browser with the URL http://localhost:3000.
user@local:~# npm run start
> nodejs-minimal@1.0.0 start /nodejs-minimal
> node index.js
minimal node.js boilerplate app listening on port 3000, open http://localhost:3000
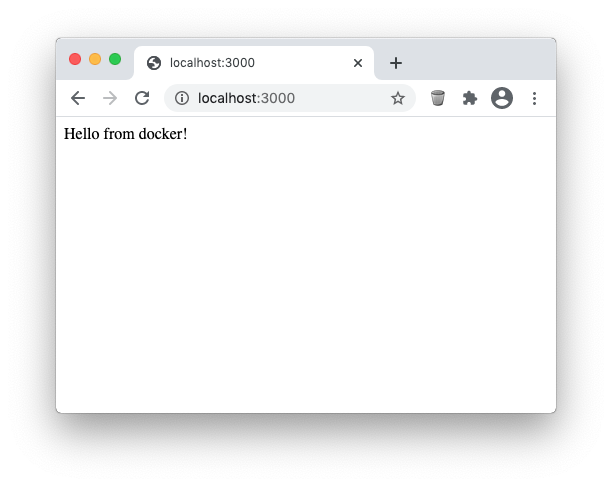
Additionally you can define the port the app will listen to by creating an environment variable named PORT:
user@local:~# PORT=3333 npm run start
> nodejs-minimal@1.0.0 start /nodejs-minimal
> node index.js
minimal node.js boilerplate app listening on port 3333, open http://localhost:3333
This is later needed to be able to make the Node.js accessible inside of Azure App Service.
2. Package Docker Image
In the next step we need to package the Node.js application in a Docker Image which can be deployed to a docker-compatible hosting service like Azure App Services. For this a Dockerfile is needed, which describes how a Docker Image should be build.
A minimal Dockerfile for a Node.js application looks like this:
FROM node:10.20.1-alpine
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY index.js ./
CMD npm start
- Using this Dockerfile a Docker Image can be built with:
docker build -t nodejs-minimal --file Dockerfile .
- and a Docker Container can locally be started with:
docker run -d -p 3000:3000 nodejs-minimal:latest
Now you can open the same URL (http://localhost:3000) as before for the non-docker running instance, and you’ll be able to access the Node.js application which now is running inside the local Docker Container:
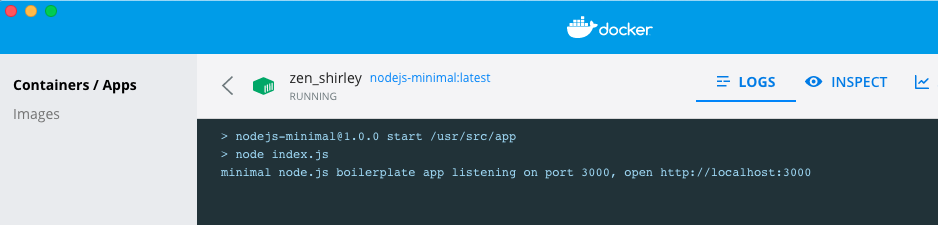
3. Create a new Container Registry in Azure & login into it locally
To be able to run a Docker Container on a hosting service like Azure App Service, we need to make our Docker Image accessible by storing it in a Container Registry. The advantage of using the Azure Container Registry is that this Docker Image can then be easily deployed inside the Azure ecosystem itself.
To create a new Container Registry login into Microsoft Azure an go to the Container registries services and create a new Container Registry:
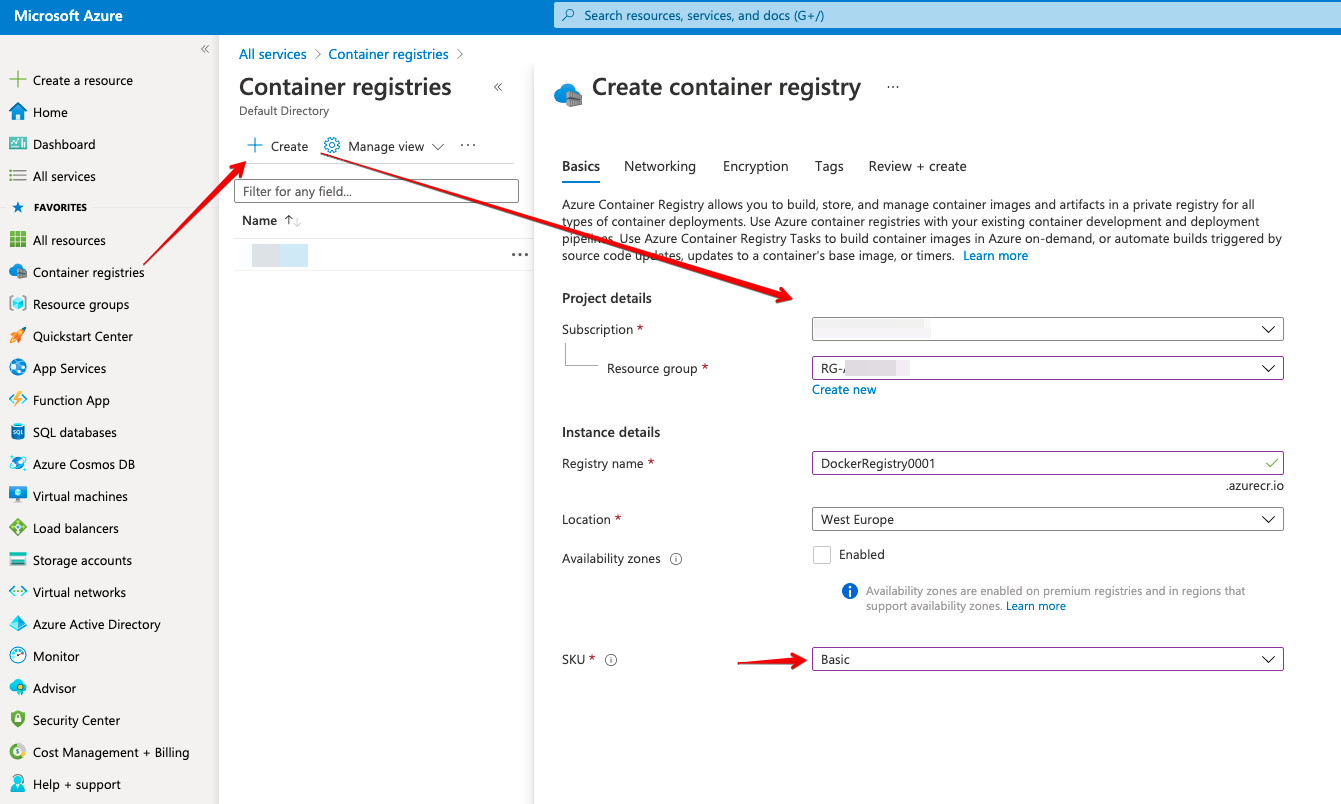
When trying out Azure services, you should use the basic SKU of the newly created container registry, which has the lowest price per hour.
After a short moment the Registry should be created and you can obtain the login data for it from the “Access keys” register after enabling the “Admin user” option:
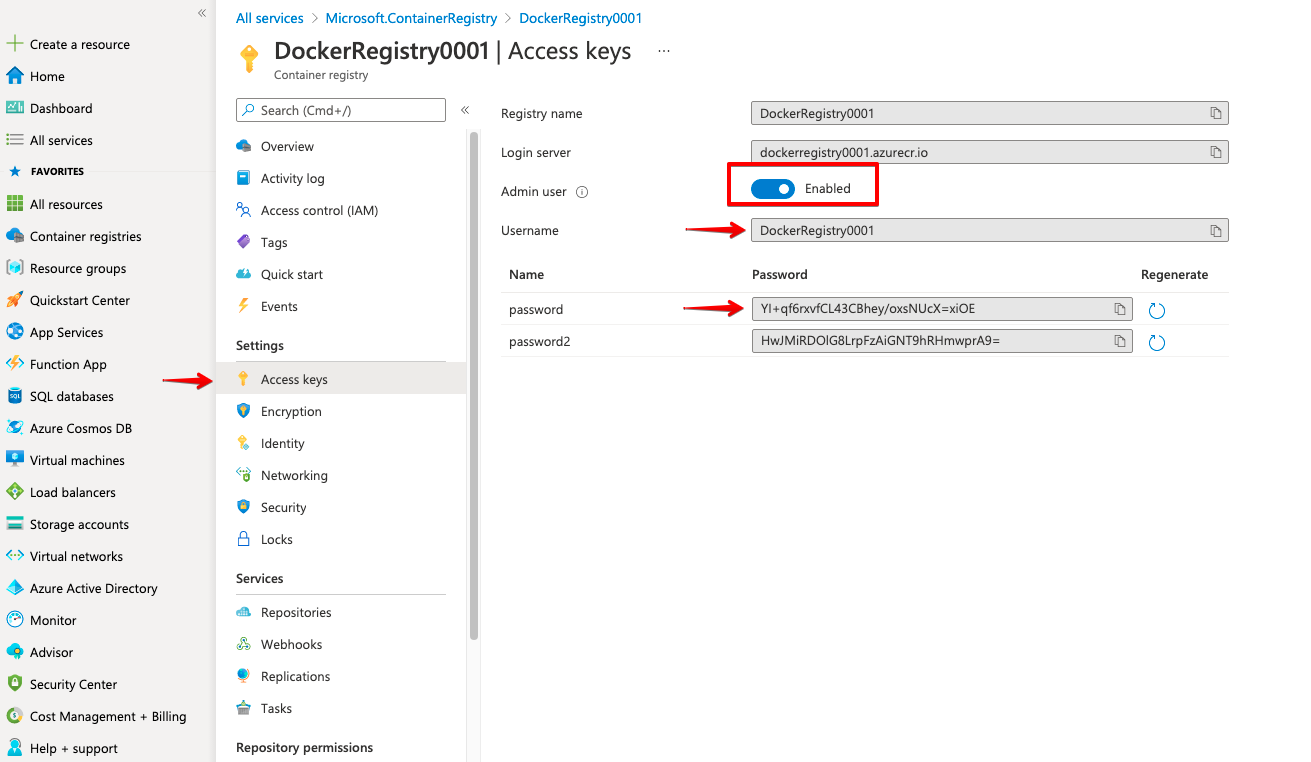
To be able to push local Docker Images to the Azure Container Registry, you first need to log in on your local machine using the docker login command & the provided access information in the “Access keys” register in Azure:
user@local:~# docker login dockerregistry0001.azurecr.io --username DockerRegistry0001 --password YI+qf6rxvfCL43CBhey/oxsNUcX=xiOE
WARNING! Using --password via the CLI is insecure. Use --password-stdin.
Login Succeeded
4. Push local Docker Image to the Container Registry
Now that we are logged in into our Azure Container Registry locally, we can push the existing Docker Image to the Container Registry. But first we need to tag our image using the docker tag command:
docker tag nodejs-minimal dockerregistry0001.azurecr.io/nodejs-minimal:latest
- Now we can push the local Docker Image to the remote Container Registry. using the docker push command:
user@local:~# docker push dockerregistry0001.azurecr.io/nodejs-minimal:latest
The push refers to repository [dockerregistry0001.azurecr.io/nodejs-minimal]
3b0091c9bfe3: Pushed
877ceef1c9d3: Pushed
15df6fb2418e: Pushed
79591984d8a8: Pushed
ed72cb3dc3da: Pushed
5b98b1e45459: Pushed
4260009d02ac: Pushed
3e207b409db3: Pushed
latest: digest: sha256:0a2b93478747faaf8bcaf8f3be63dab289326524b1427f6a02ab19421b360002 size: 1991
If the docker push of to the local Docker Image was successful, you’ll see it listed in the Azure web interface of the Container Registry:
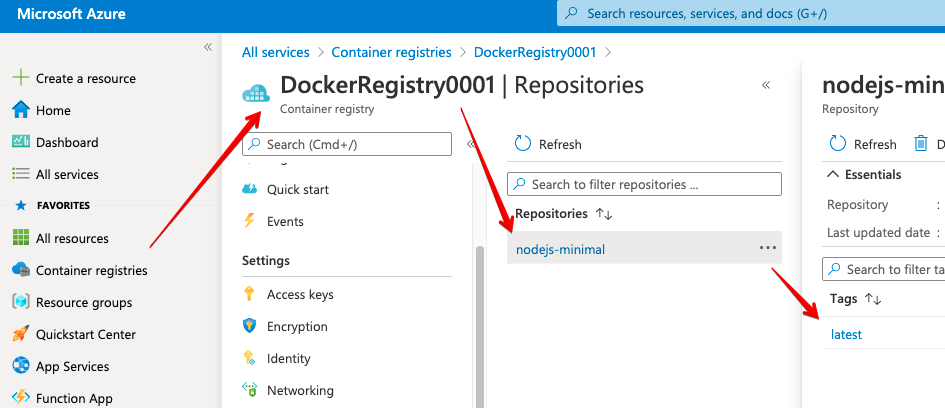
5. Deploy Docker Image to Azure App Services
Now that Docker Image has been uploaded to Azure, it can easily be deployed inside existing Azure hosting tools, like the Azure App Services.
For this go the the Container Registry Docker Image list and deploy the Docker Image as a web app to an existing or newly created Azure App Services Plan:
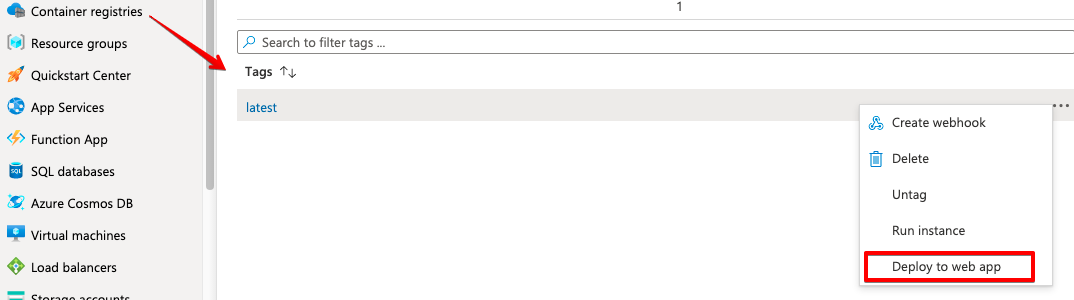
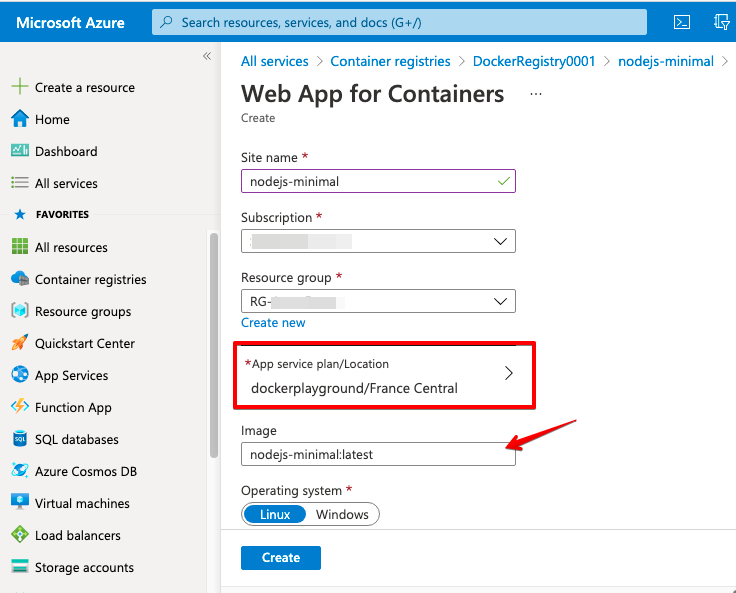
After a short while the deployment of the web app should be finished and the application can be accessed by opening the public URL shown in the App Service window which can be reached from the “Apps” register tab of the hosting App Service Plan:
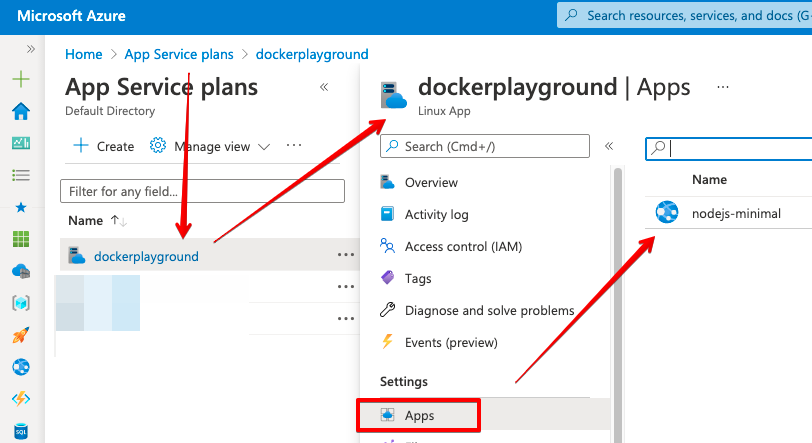
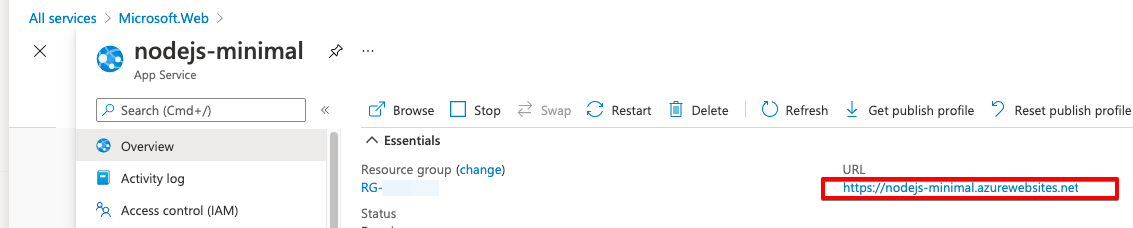
Conclusion
In this article we went from creating a minimal, locally running Node.js app. Then this app was packaged into a Docker Image which in turn has been uploaded to an Azure Docker Registry and in the last step deployed as a web app inside of Azure App Services.
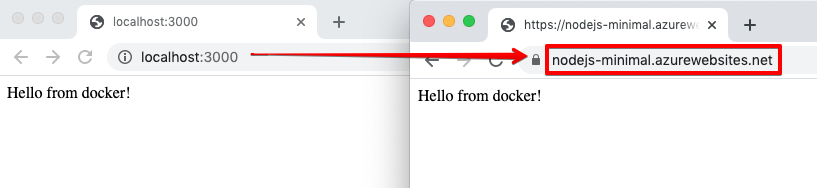
↑ back to top ↑